Beginner's guide to creating a GNOME 2 applet with Python (Part II)
Posted on 19 August 2009 in Articles • 4 min read
Part II: GNOME applets and PyGTK
Before we begin...
If you have read part I of the tutorial, and have a test applet running in both "panel" and "debug" mode, you would have no problems running all the code described below. All terms described in part I, keep their meanings here so, if you have not read the article, you can do it now.
1. Launching an applet
Generally, a GNOME applet could be treated as an application "attached" to the panel through Bonobo component model system [2]. We can launch the sample applet in a standard GNOME window with the -d key [1]. Yet, for example creating and attaching a menu to an applet is not the same task of creating a menu for a windowed application. Or, an applet will always be vertically-oriented" in debug-mode. So, this time the applet will be added to a panel during all test-runs.
Note
If the sample applet doesn't appear on a panel, it means, that something went wrong (a possible bug in a script). Try launching the applet in debug mode to see the output and catch possible bugs.
3.1 Applet's orientation
An applet could be set up both on a horizontal or vertical panel. This should be taken into account before creating the visual elements of an applet. Also an applet's orientation could be changed by user (dragging an applet from a horizontal panel to vertical panel), in this case a callback for the change-orient signal should be created [5].
1 2 3 4 5 6 7 | def change_orientation(applet, orient, user_data): pass def applet_factory(applet, iid): applet.connect('change-orient', change_orientation) ... ... |
The code below demonstrates how change-orient signal could be used:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | def applet_factory(applet, iid): # initialize an orientation-dependent label orientation = applet.get_orient() if (orientation == gnomeapplet.ORIENT_UP or orientation == gnomeapplet.ORIENT_DOWN): label = gtk.Label("Vertical") else: label = gtk.Label("Horizontal") # label is the user data applet.connect('change-orient', change_orientation, label) ... ... def change_orientation(applet, orient, user_data): orientation = applet.get_orient() label = user_data if (orientation == gnomeapplet.ORIENT_UP or orientation == gnomeapplet.ORIENT_DOWN): label.set_label("Vertical") else: label.set_label("Horizontal") |
The applet was added to a vertical panel:
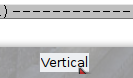
Then, dragged to a auto-hidden horizontal one:
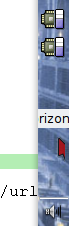
3.2 Applet's background
The GNOME panel has three background options:
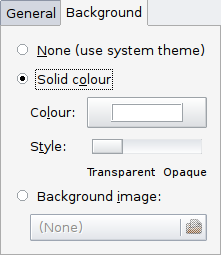
And an applet can detect these options' changes via the change-background signal [6].
But in practical situations, an applet doesn't respond to the panel's background changes. What really matters is an applet's background transparency. This is where the power of GNU helps: the sources of GNOME trash applet contain a "hack" line of code that makes an applet transparent. In Python it is:
1 2 3 4 | def applet_factory(applet, iid): applet.set_background_widget(applet) # /* enable transparency hack */ ... ... |
Now, the applet should look like this:
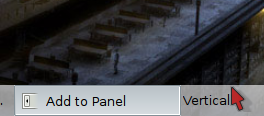
That's it! This is the end of the second part of the tutorial. There is not much left to say about the applet techniques. But there is much to learn about PyGTK (creating dialogs, different widgets, signals, etc.). A great tutorial is available at the official site http://www.pygtk.org.
References
[1] | Beginner's guide to creating a GNOME applet with Python (Part I) |
[2] | Bonobo component model |
[3] | The PanelApplet object |
[4] | GTK stock items |
[5] | www.pygtk.org - GNOME applet with python |
[6] | The "change-background" signal |